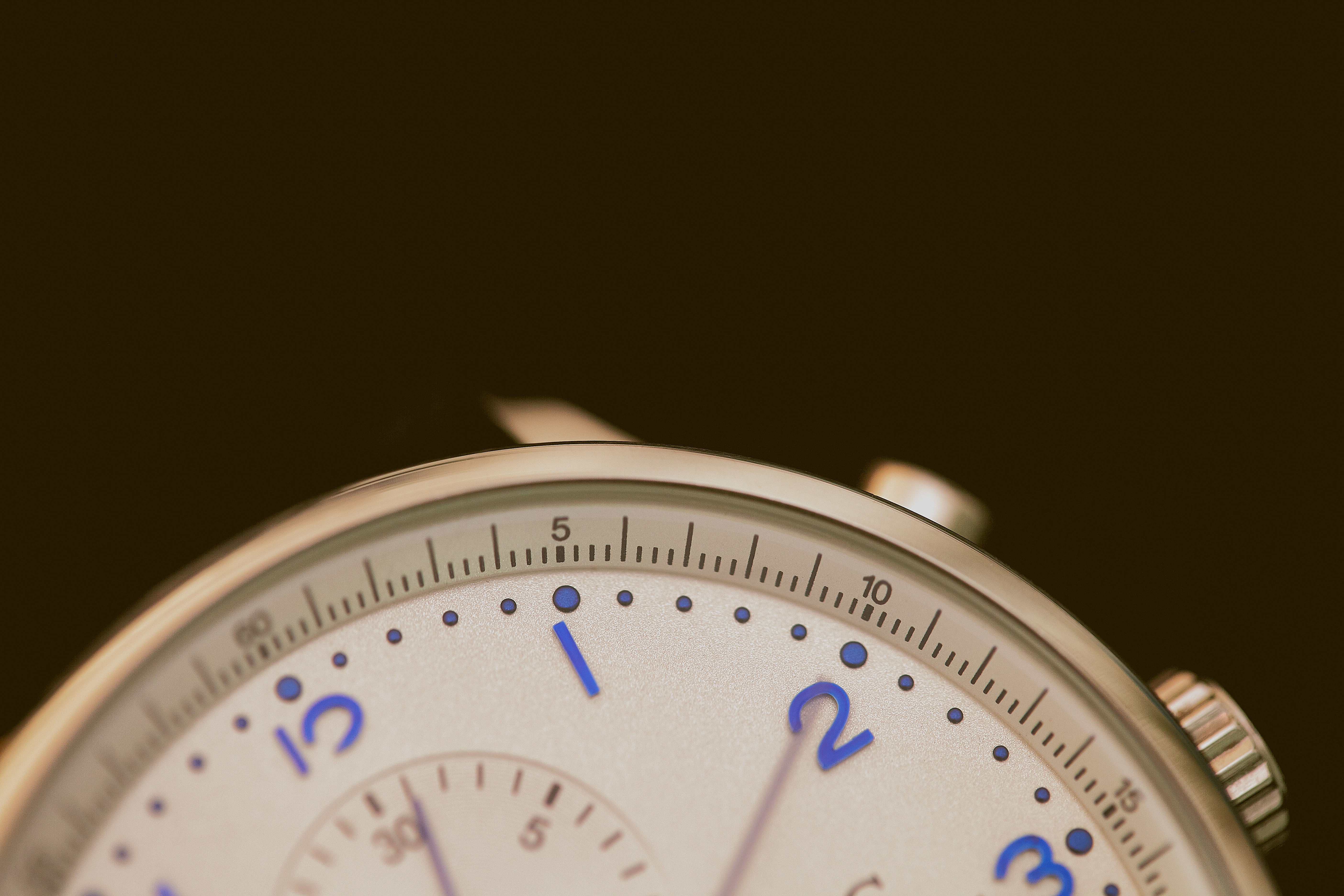
When it comes to how my brain works, there are a couple of related struggles that I’ve known about for many years and that I’m finally going to do something about.
Regularly I’ll stare at a computer screen for far longer than the task should require, making slight changes to a document, or an email draft, or some code, failing to fix the broken thing.
When working in a team I would likely find myself asking for support from others, or I would hope to be asked how I was getting on. I need to police this myself when working remotely and on a solo task.
I’ve previously tried making use of blocks or time. Pomodoro 25 minutes for example, which encourages breaks in focus to take a minute, a breath and a walk. Specific amounts of time haven’t worked for me, but prompts at regular intervals to consider a break have a more positive effect.
I see it as a three strikes rule. If I see a prompt once, I’ll happily keep plugging away. Two prompts and I start to justify to myself that I know how to get it resolved relatively quickly. When the third prompt appears I have to call my own bluff and take a break. The break needs to be a real break away from a screen thinking only with my brain and not with my eyes. Thinking in a different way, or maybe not thinking about it at all.
This change of focus, or bluring of focus, allows my brain to either solve it ready for my return to my computer, or when I do return to my work my brain is in a better place to make progress.
The other change I’ve made is how I handle the beginning and end of a day during which I don’t commute to a meeting or client.
A commute is a really useful tool when it comes to preparing for being in a work or home environment. On days where I’ll be at home in the morning preparing for work, and then suddenly find myself working, I stuggle to stay focused. On days where I’m working at home, and the only thing that happens between work and family time is a walk down the stairs, I struggle to switch off and engage with other humans.
I need the boundaries to be more defined.
For some I imagine this can be achieved by taking kids to school, walking the dog or even a short chunk of exercise. For me it can be as simple as walking slowly to the end of the road and back. It also helps with those still in the house to understand that on return I’m in work mode.
If I’m not commuting then I need this line in the sand both in the morning and at the end of the day.
The context switching or decompression time between home and work tasks is vital to allow me to be engaged with the right thing.